by admin | Mar 10, 2016 | 2016 |
Most awaited feature for Web application automation is implemented in QTP 11.
Yes, it is Running JavaScript snippets during run-time. It is really helpful to trigger a JavaScript function which is attached to an HTML object.
Code
Dim oPage:Set
oPage=Browser("name:=Google").Page("title:=Google")
Dim oTxtGoogle:Set
oTxtGoogle=oPage.RunScript("document.getElementsByName(
'q')(0);")
'The above line gets DOM object for Google textbox
using Javascript.
oTxtGoogle.Value="qtp"
by admin | Mar 10, 2016 | 2016 |
If you want to retrieve cursor position from an WebEdit, it can be done using Range boundary from Selection.
Code
Dim oPage:Set oPage=Browser("name:=Google").Page("title:=Google")
Dim oSel:Set oSel=oPage.Object.Selection.createRange
Dim oWebEdit:Set
oWebEdit=oPage.WebEdit("name:=q").Object
oSel.moveStart "character",-Len(oWebEdit.value)
Msgbox Len(oSel.text)
'Here you will get the cursor position.
Set oPage=Nothing
Set oSel=Nothing
Set oWebEdit=Nothing
by admin | Mar 10, 2016 | 2016 |
Since this is the very first article in this blog, we would like to start it with well known
masking input value technique using Dot Net Factory.
Code
Dim oForm, oTextBox, oCloseButton, oPoint, inX, inY
Set oForm=DotNetFactory.CreateInstance("System.Windows.Forms.Form","System.Windows.Forms")
Set oTextBox=DotNetFactory.CreateInstance("System.Windows.Forms.TextBox","System.Windows.Forms")
Set oCloseButton = DotNetFactory.CreateInstance("System.Windows.Forms.Button", "System.Windows.Forms")
Set oPoint = DotNetFactory.CreateInstance("System.Drawing.Point", "System.Drawing", inX,inY)
With oPoint
.X=180
.Y=33
End with
With oCloseButton
.Location = oPoint
.Text = "Close"
End with
With oPoint
.X=10
.Y=35
End with
With oTextBox
.Location = oPoint
.Width=160
.UseSystemPasswordChar=True
End with
With oForm
.Height=120
.width=275
.text="Masked Inputbox"
.CancelButton = oCloseButton
.Controls.Add oCloseButton
.Controls.Add oTextBox
.MaximizeBox=False
.MinimizeBox=False
End with
oForm.ShowDialog
Msgbox oTextBox.Text
Set oForm=Nothing
Set oTextBox=Nothing
Set oCloseButton=Nothing
Set oPoint=Nothing
Synopsis
After executing the above code, you will get Input Dialog as shown below. Enter value in the textbox control and click on Close button.
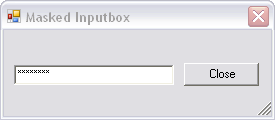
by admin | Mar 10, 2016 | 2016 |
Using QTP we can capture an object or Desktop using CaptureBitmap method. How to capture a region of screen?
It can be done using System.Drawing.Graphics.CopyFromScreen method. Let us see how it can be done using this method.
Code
'Function Name: CaptureRegion
'Argument 1: strPath- File path where you want to store the captured image.
'Argument 2: inX- X co-ordinate
'Argument 3: inY- Y co-ordinate
'Argument 4: inHeight- Image Height
'Argument 5: inWidth- Image Width
Function CaptureRegion(ByVal strPath,ByVal inX,ByVal inY,ByVal inHeight,ByVal inWidth)
'Declaring all the required Local variables
Dim oBitmap,oPixelFormat,oGraphic,oPoint,oSize
'Creating Pixel Format object
Set oPixelFormat=DotNetFactory.CreateInstance("System.Drawing.Imaging.PixelFormat","System.Drawing")
'Point structure to specify x and y to capture a region
Set oPoint=DotNetFactory.CreateInstance("System.Drawing.Point","System.Drawing",inX,inY)
'Size structure for new image
Set oSize=DotNetFactory.CreateInstance("System.Drawing.Size","System.Drawing",inWidth,inHeight)
'Creating Bitmap object with Height, Width and Format32bppArgb pixel format
Set oBitmap=DotNetFactory.CreateInstance("System.Drawing.Bitmap","System.Drawing",inWidth,inHeight,oPixelFormat.Format32bppArgb)
'Graphic object
Set oGraphic=DotNetFactory.CreateInstance("System.Drawing.Graphics","System.Drawing")
'Mapping Bitmap object with Graphic object
Set oGraphic=oGraphic.FromImage(oBitmap)
'Capturing the screen region using CopyFromScreen method
oGraphic.CopyFromScreen oPoint,oPoint,oSize
'Saving the file
oBitmap.Save(strPath)
Set oPixelFormat=Nothing
Set oPoint=Nothing
Set oSize=Nothing
Set oBitmap=Nothing
Set oGraphic=Nothing
End Function
Dim strPath
strPath="C:regionCapture.png"
'Bringing the screen to be captured to foreground
Browser("name:=iGoogle").Page("title:=iGoogle").Highlight
'Calling CaptureRegion function
Call CaptureRegion(strPath,0,0,20,40)
Synopsis
This technique is very useful as our testing might require capturing region of a screen. Keep visiting for more imaging techniques articles.
by admin | Mar 10, 2016 | 2016 |
.Net Regular Expression engine is more powerful than VBScript Regular Expression engine. RegEx is one of the classes of .Net Regular Expression engine. Using the Split method available in RegEx class, we can split a string with Regular Expression pattern. Let us see the implementation below.
Code
Dim oRegex,oArrayList
'Creating instance for RegEx
Set oRegex=DotNetFactory.CreateInstance("System.Text.RegularExpressions.Regex","","")
'Creating instance for ArrayList
'this is required to hold the elements since Split method returns String array.
Set oArrayList=DotNetFactory.CreateInstance("System.Collections.ArrayList","")
'Splitting the string with Regular Expression
Set oArrayList=oArrayList.Adapter(oRegex.Split("quick test professional", "W+"))
'Displaying the elements
Msgbox oArrayList(0)
Msgbox oArrayList(1)
Msgbox oArrayList(2)
Set oRegex=Nothing
Set oArrayList=Nothing
Synopsis
This method is very useful whenever we need to split a string with Regular Expression pattern.
Note: Since ‘RegEx’ Split method returns String array, VBScript Array cannot hold the elements which are returned by this Split method.
by admin | Mar 10, 2016 | 2016 |
Sending an email using VBScript can be done using Collaboration Data Object (CDO) messaging. But it is supported only in Windows 2000, Windows Server 2003, Windows Server 2008, Windows Vista, and Windows XP Operating Systems. To create object for CDO, cdosys.dll should be installed in your machine and many users reported failure when CDO is implemented in Windows 7. Then what is the solution for this problem?
We have an alternative, which is System.Net.Mail .Net class. Microsoft introduced this class when .Net Framework 2.0 was launched. The following are the major benefits of Net.Mail class,
- CDOSYS.dll installation is not required to send an e-mail.
- More powerful than CDO messaging.
- Attaching file with message is very simple like adding recipients.
- Attachment can be done for a filename or a stream.
Code
Dim strUserName,strPassword,strServerName
Dim strFromAddress,strToAddress,strFileToAttach
Dim oCredentials,oSMTPClient,oMessage,oFromAddress,oAttachment
'Setting Username
strUserName="Username"
'Setting Password
strPassword="Password"
'Specify SMTP Server name or IP address of the host computer.
strServerName="smtp.gmail.com"
'From Address
strFromAddress="[email protected]"
'To Address
strToAddress="[email protected]"
'File which will be attached to message
strFileToAttach="C:TestResult.html"
Set oCredentials=DotNetFactory.CreateInstance("System.Net.NetworkCredential","System",strUserName,
strPassword)
Set oSMTPClient=DotNetFactory.CreateInstance("System.Net.Mail.SmtpClient","System",strServerName)
Set oMessage=DotNetFactory.CreateInstance("System.Net.Mail.MailMessage","System")
Set oFromAddress =DotNetFactory.CreateInstance("System.Net.Mail.MailAddress","System",strFromAddress)
Set oAttachment=DotNetFactory.CreateInstance("System.Net.Mail.Attachment","System",strFileToAttach)
'Set ‘From’ property to Message object
oMessage.From=oFromAddress
'Set ‘To’ property to Message object
oMessage.To.Add strToAddress
'If your message has to be formatted with HTML, then make this property value as True.
oMessage.IsBodyHtml=False
'Email Subject
oMessage.Subject = "Test Mail"
'Email Body
oMessage.Body = "Automated Email"
'Adding the oAttachment object to Message object
oMessage.Attachments.Add(oAttachment)
'Setting PORT 587 for outbound mail
oSMTPClient.Port = 587
'Setting Credentials using NetworkCredential class
oSMTPClient.Credentials=oCredentials
'Enabling Secure Socket Layer (SSL) to encrypt the connection
oSMTPClient.EnableSSL = True
'And finally sending the message
oSMTPClient.Send(oMessage)
Set oMessage=Nothing
Set oFromAddress=Nothing
Set oCredentials=Nothing
Set oAttachment=Nothing
Set oSMTPClient=Nothing
Synopsis
System.Net.Mail class is not dependant upon COM and it is more versatile than CDO messaging.
Note: We can also embed an image into the message using LinkedResource class.
Reference: http://www.systemnetmail.com/