by admin | Nov 16, 2019 | Automation Testing, Fixed, Blog |
Test automation reporting is an important component in the automation framework. Once your automated test suites are executed, test results will be the only artifacts to analyze failures. You will be forced to execute the failed test cases to investigate further if you don’t report the failures properly. As a test automation services company, we use automation testing dashboard reporting tools to report the results and logs. In this blog article, we have listed a few key test automation reporting tools which are widely used by automation testing communities across the globe.
Perfecto – Smart Reporting
This is a commercial automation testing reporting tool from Perfecto. Smart reporting is implemented with AI-Powered algorithms which provide the insights you need and reduce false negatives for less noisy reporting. This is the best Visual Analytics Tool for Test Reporting.
Calliope
Calliope.pro is a DevOps tool that generates comprehensive test results. You can also publish the results from Cucumber, TestNG, and JUnit. This is also a paid tool and its web dashboard allows your team to visualize and analyze the test results.
Dashing.io
Dashing is a dashboard framework. If you would like to customize your dashboard report and are not interested in paid or other predefined test automation reporting tools, then Dashing.io will be the right choice for you. You can build a dashboard using JSON output from test automation execution.
Allure
Allure is an open-source framework designed to create test execution reports that are clear to everyone in the team. It has client libraries for the following programming languages – Java, PHP, Ruby, Python, Scala, and C#.
Extent Reporting Framework
With Extent Framework, you can create beautiful, interactive, and detailed reports for your tests. Add events, screenshots, tags, devices, authors or any other relevant information you decide is important to create an informative and stunning report.
ReportPortal.io
ReportPortal web dashboard provides AI-powered automation testing report. You can manage all your automation results and reports in one place and submit & track defects with full evidence (logs, screenshots, comments, etc.) directly from a failed test case into a bug tracking system.
by admin | Nov 27, 2019 | Automation Testing, Fixed, Blog |
The impact of the disruption in the Test Automation space in the Software Testing (ST) industry is enormous this year. Due to the emerging Artificial Intelligence (AI) solutions, automation tool self-healing is a possibility as tons of data collected for every test run is fed into the device’s machine-learning algorithm. A transition towards Machine Learning (ML) will help report automated test runs and analysis. New automation-based tools will gain popularity, and Selenium WebDriver, the de facto browser-based automation tool for testers, will slowly be replaced. Testing organizations need to use the right tool for the right job in their development pipeline.
AI-based automation testing options introduced right now are paid solutions, and TestCafe, Cypress.io, and Jest are some examples. Although it is a contradiction since WebDriver is a W3C standard, the latest AI-based tools have record and playback functionality and use ML to help improve reliability during runtime. Continuous testing (CT) is the catchphrase now, and we can define it as the ability to instantly assess the risk of a new release or change before it affects customers. By implementing CT methodologies, automated tests are executed to check the quality of the software during each stage of the Software Development Life Cycle (SDLC).
Vendors are creating end-to-end testing solutions for their clients, and they need to acquire tools that aren’t part of their existing catalog. Performance testing or test management is causing more mergers/acquisitions this year. A huge collaboration is evident since the market is demanding investment in testing tools for businesses to develop quality software.
Let’s move on and break down some of these ST trends that will take over the industry.
Test automation services companies have promoted current software releases at optimum quality while decreasing ordinary testing efforts. The Codeless Test Automation tool increases scalability and facilitates software testers or business users to automate their test cases without worrying about the coding.
ST is when applications under specific conditions are tested to identify the risks involved in software implementation for greater automation to ensure optimal accuracy in the goal towards digital transformation. The industry is using AI to make such applications reliable and signify a shift from manual testing, and human interference towards a movement wherein machines gradually assume control.
Enhancements to AI and ST pave the path for Robotic Process Automation (RPA). Amongst transforming technologies like the Internet of Things (IoT), AI, ML, and Cognitive Computing, RPA is the latest entrant with the ability to re-invent the entire business process management aspect in this industry.
The demand to adopt new changes in the industry has resulted in an upgrade to practices and methodologies like DevOps and Agile. The QA team needs to plan and execute test strategies, provide quality products through continuous testing, making them accountable. Even complicated systems can drive faster deployments, assure optimum quality, and deliver cost-effective outputs.
QA Automation Services Companies have embraced IoT apps and devices to test performance, security, and usability to make technological improvements within the ST industry. Push testers and QA teams enhance their skill set regularly through analysis and modifications. The time-to-market of your product can make all the difference between success or defeat as users are becoming more selective, especially when it comes to the quality of the solutions.
That’s why at Codoid, our mantra is to ‘stay up to date with the times’ because, as an Automation Testing Services Company, we are continually upgrading our systems and streamlining processes while testing new developments in the industry. It helps us guarantee the best results for our clients, so don’t hesitate to pick up your phone and give us a ring. Let us help you bring forth solutions to catapult your business forward.
by admin | Nov 17, 2019 | Automation Testing, Fixed, Blog |
The benefits of test automation are widely known – delivering high-quality software and solutions speedily and cost-effectively. Automating tests is about assuming that the tests will be conducted repeatedly and with fewer errors. Despite these benefits, it is necessary to remember that automation of tests needs careful consideration prior to implementation owing to the time and financial investment required.
Working with a professional Test Automation Services Company will help to answer your questions and would consider several aspects before suggesting automation of tests. As experts, we will help define the requirements, put together goals, assess ROI, and ensure complete clarity for all stakeholders. We at Codoid, have put together a list of considerations required prior to automating tests.
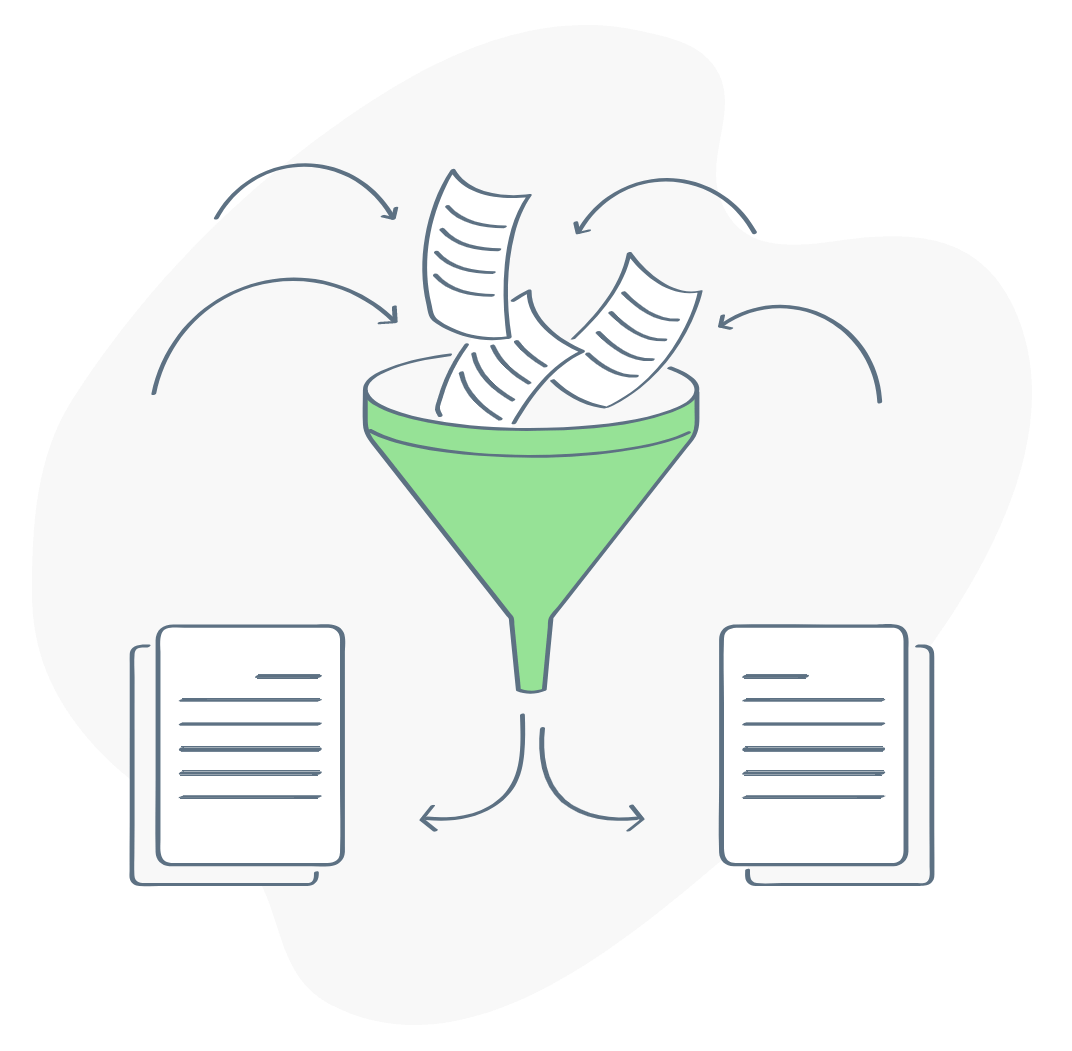
- When a product has several new releases and defect correction in a running module, automation becomes necessary to check that the bug corrections have not introduced other bugs. The previous functionality would need testing as well, and doing this repeatedly manually would not be an efficient or effective method. The costs in terms of resources, time, and effort would not justify manual testing.
- It is far more effective to automate the tests for web applications that would have a very large number of users simultaneously interacting with the application. Creating the users manually would be extremely tough and time-intensive.
- Automate tests if there are frequent functional changes and GUI is almost static.
- Check the risks of automated testing prior to switching tests to automated mode. As an expert Automation Testing Services Company, we understand the many challenges and risks associated with test automation. Lack of skilled in-house resources is a major risk. Without personnel with programming knowledge, technical abilities, and the skill to adapt speedily to new technologies, automating tests could prove damaging. While manual testing requires investment to hire top-quality manual testers, automation has its share of costs. The initial in-house setup costs including buying automation tools, training the staff, and also maintaining test scripts are quite steep. Several companies now decide in favor of a highly skilled and professional partner offering Automation Testing Services, which proves cost-effective and mitigates the risks of software automation testing.
- Basic User Interface (UI) automation suffices if it is constantly changing. To automate a consistently changing UI would mean incurring a very high cost for script maintenance. As experts, we do not recommend this.
- Unless using an agile environment, it is not recommended to switch to automated testing in the early stages of SDLC since the costs will be prohibitive.
- Complete or 100% automation is not feasible or possible. There are several areas such as testing for compatibility, installation, recovery, UI, documentation – which must be tested manually.
- There are several tests that only require to be run once, and hence would not be a part of regression. Such tests/modules must not be automated.
- The automation suite should be usable or run about 15-20 times each for individual builds in order to pose a high ROI.
In Conclusion
While automation testing is the top method to achieve most of the objectives of testing, and is also the most effective use of time and resources, it is important to remain aware of the considerations of automating tests. It is not a wise move to expect unskilled and inexperienced staff to manage the expensive and comprehensive automation tools. Handling these tools is best left to the experts. We at Codoid recommend a combination of both manual and automation testing to achieve top results for projects. Connect with our experts to experience the benefits of both types of testing and who will ensure that your products gain the highest ROI for you.
by admin | Nov 1, 2019 | Automation Testing, Fixed, Blog |
Planning and strategy are the cornerstones of the SDLC and the main aim of testing should be to ensure that business requirements are met and the customer receives a product with zero defects. As a high-quality software testing company we consistently strive to meet this aim, gaining for us a wide base of happy and long-term customers. An effective test automation strategy requires some serious planning and in-depth expertise.
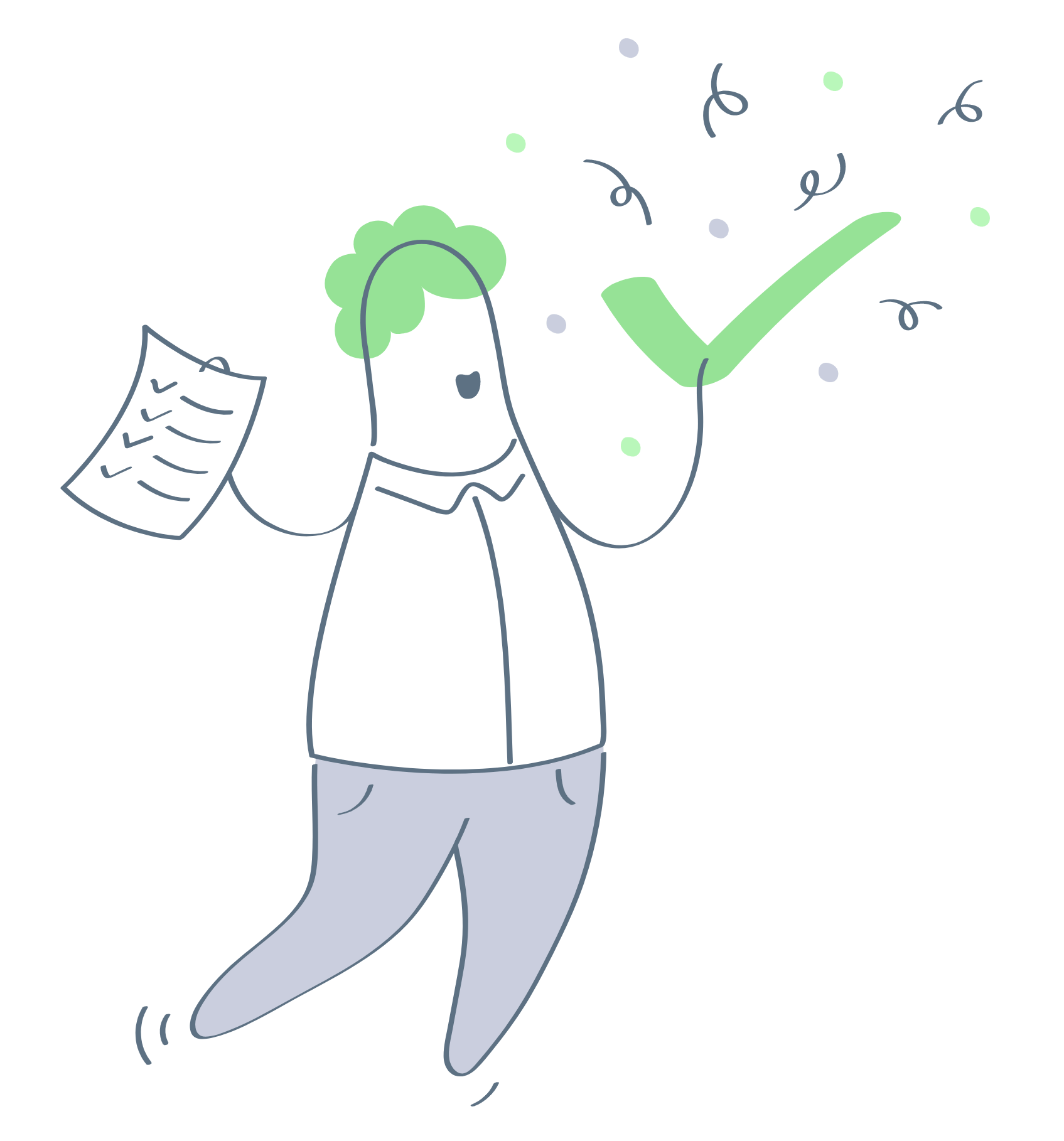
Even the most efficiently run business needs an efficient automation testing company partner to ensure that testing exercises run smoothly and the end product is defect-free – we are the go-to partner. We are experts in the realm of Automation Testing Services with experience in building cost-effective automation testing strategies for our clients, with result-orientation. We know that the absence of an effective test automation strategy, or even a poorly constructed one, would be the cause of testing failure. This in turn would lead to poor user experience, causing major losses both in terms of money and reputation of a company.
How Critical to Success is a Test Automation Strategy?
With the growth in market challenges and customer demands, software testing has undergone some significant transformations. Businesses want applications and software that can withstand pressures, including customer expectations, the risk of bugs, and security threats. Agile and DevOps approach ensures that testing is done regularly and thoroughly – forming a critical part of the overall test automation strategy. As experts in the realm of Test Automation Services and more, we understand the use of this approach to make the testing process faster, repeatable, and leading to a high-quality application.
Most appropriate test automation tool selectionThis is the main component that can guarantee the success of a test automation strategy. There are several tools in the market, and the ones we select are based on the testing and feature requirements. We consider parameters such as maintenance, the cost of the tool, training, and support required, the performance and stability of the tool, and more. Our clients gain peace of mind in addition to seamless testing and top-quality product.
Establishing Test Automation EnvironmentAfter identifying the needs of the test environment and acquiring tools and licenses, the test environment must be set up – replicating the production environment. This essentially implies that the configuration on each server of both hardware and software will be the same.
Automation Scripts must be ReusableThe code or script and framework should be reusable across teams at an organizational level. This reduces the time and effort required to develop scripts in the future.
Meticulous Execution and Management of Test CasesThe strategy must include a well-defined process to execute and manage the test cases, as per the defined criteria for success.
Result-OrientationThe results of each run of test automation must be measurable and have a result orientation. The test metrics of varying KPIs and dashboards would ensure the quantification and qualification of the software and highlight the standards of quality of the software before launching to the market.
Capture Learning in DocumentationIn the course of software development there would be several things that should be captured as learning for the future. It is important to put together robust documentation to ensure that future test automation strategies would be better and improved – especially not repeating mistakes from the past.
In Conclusion
As testing experts we can help businesses by recommending tools and techniques that would guarantee top results. As a testing partner, we prevent high maintenance, cost, and time by consistently revisiting and updating the automation test suites based on the previous latest version – over time. Since we are the experts, it is easy for us to run this as a continuous activity, allowing us to remove obsolete test cases, and regularly upgrade the automation suite with new functionalities. We understand the importance and practicability of setting short-term goals that are revisited periodically to ensure an iterative incremental model, which would lead to a robust and effective test automation strategy. Connect with us to know more about our expertise.
by admin | Apr 30, 2020 | Automation Testing, Fixed, Blog, Top Picks |
The decision of setting up Automation CoE has to come from QA head of a software testing company and he/she should have a compelling need to setup this Organization.
Discussion with different Business Units to understand the landscape of Applications, life span of Application in-scope, different technologies involved, current QA tool stacks, how much of automation is achieved till date and how much it is benefiting to them in the current state and future benefits prediction has to be analyzed.
If we have this details in place, it would help us to quantify the amount and nature of work involved and help in analyzing the ACoE (Automation Center of Excellence) team size.
The above requirement would be for Product based companies. If it is a service-based company, below are the requirement to setup ACoE team.
1. Collate the history of at-least 1 year on overall Automation requirements from Customer
2. Nature of Customer and their maturity level in Automation
3. Level of interest in Automation and Automation investment criteria
4. Nature of projects like Staff Aug,
5. Type of requirements they have
Could be identifying opportunity within Customer Organization
Tool and Framework assessment
Training activities
This will give us an insight on how to start an ACoE team from the service companies perspective.
Conclusion:
Since we are in the world of digitalization, there is always a great demand for Automation in the future beyond traditional Automation. We should prepare ourselves for such changes that the industry is undergoing and flow with the tide. The expectation from such Center of Excellence is also growing and we should prepare ourselves for these changes.
by admin | Oct 26, 2019 | Automation Testing, Fixed, Blog |
As a business you would constantly be mulling over reducing testing and automation costs, albeit without conceding or making concessions on the quality of your product. Those who may have erred would understand the disastrous consequences for their business with regard to poor quality products resulting from shoddy software testing. As a leading Test Automation Company we know the major overhaul required post-release for applications and software in order to save company reputation and prevent loss of user loyalty. Companies spend big monies on allocating a large number of resources for software projects, and yet there are no guarantees that the ROI would justify these humongous expenses. On the other end of the spectrum, some companies allocate too few resources, which too prove detrimental to the ‘health’ of envisaged the software/application.
It is, therefore, necessary to find a perfect balance of time, costs, and effort, and it makes sense to partner with a company experienced in delivering top-class automation testing services within budgets and timelines. We enlist some ways to bring down the costs of testing and automation without any compromise on the quality and output of the software.
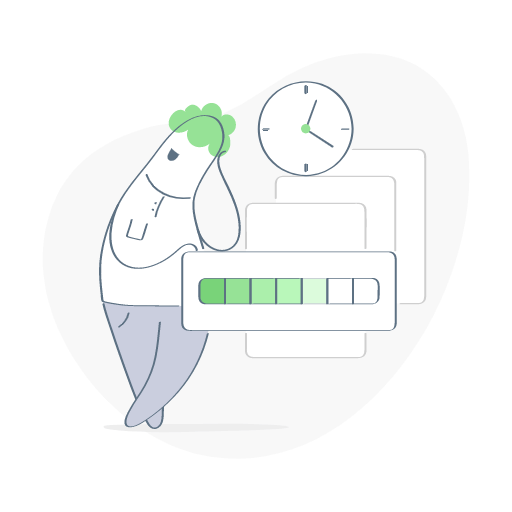
Testing as Early as Possible
As an experienced Automation Testing Company we always recommend testing products early on in the SDLC to avoid problems and errors close to release or post-release. It is important for the QA and development teams to work cohesively and in collaboration in order to reduce the possibilities of production errors. It stands to reason that the earlier errors are identified the cost of rectifying them would be a lot lower than the costs for the ones discovered post-release – in fact in our experience, fixing defects and bugs at a later stage costs at least 30 times more than doing so at the prototype stage.
Testing Strategy must Balance Costs and Benefits
Most companies make the costly mistake of assessing efficiency by way of test coverage versus cost. This is incorrect since test coverage, is not an efficient metric – low test coverage is mostly a cause of problems, while high test coverage may not actually mean anything. Right test coverage is important and it is possible to know what this through simple methods. The production stage should rarely have bugs and changing code would not be a feared activity – these are two criteria that make the right test coverage. It is important that only the optimal time and resources are spent on test coverage, and hence both teams (development and QA) must work together to ensure the best test coverage, with minimal errors and costs.
Outline Goals and Expectations
An experienced testing partner will ask relevant and suitable questions in order to understand the product quality required by a business. The answers to these questions would serve as the foundation and stepping stones to ensure optimal and top-class product quality. The goals and expectations should get answered through the right questions, based on which the testing team would be able to put together some key performance indicators. These should include the types of testing, milestones and due dates of testing, possible risks, and challenges. An experienced testing team will ensure balanced and wise automated testing such that time, money, and effort are used minimally.
Quality is Better than Quantity
Partnering with an experienced, expert, and skilled company would get you all the services required within a budget and timelines, and higher quality than you may believe possible. It does not make sense to hire expensive human resources to handle projects that may or may not be qualified to understand the needs and requirements of the business. We have helped several clients with their projects, achieving a high level of success through ensuring top-quality products consistently. Outsourcing to us as the QA experts will get you all the benefits your project deserves.
In Conclusion
As experts, we endorse the view that testing and automation are the cornerstones for high quality applications and software. Yes, everything requires an investment of time, money, and effort, and it is imperative for a company to weigh the costs against the benefits of test automation. Enlisting the services of professionals will ensure that your business will get the best strategy and coverage for the project in question and for future projects as well. As a skilled company we offer – the use of the best tools, ways to hasten the process, remain mindful of possible challenges and bottlenecks, make the process easier and more accessible, keep to timelines, and most importantly keep costs to the minimum – all of this with top-class customer service. We at Codoid provide the Best Automation Testing Services to stride ahead of your competition and to ensure that your products continue to be loved and used by your target audience.